How to Fork This Site
How-to on forking this site from github, customizing the content for your own portfolio, and deploying the site yourself.
The goal of this site is to share my work, create a portfolio, and to have a platform for my writing outside of a platform like Linkedin, Medium, or Substack. I initially wanted to make this site available to others to use as a portfolio, but I have made many changes and want to keep my site separate. Thankfully, this is a fork of another site by Jolly Coding, and I can outline how to make changes to that site to achieve the level of personalization you are looking for.
After close to a year and a half of messing around with websites and trying to develop a platform for my portfolio, I am happy with how this one turned out, and think anyone looking to self-publish their works would find this site useful.
About the Site
This website is a fork of Jolly Coding's Jollyblog site from his NextJS 14 Blog tutorial. It is built with NextJS, MDX (which we will cover more later), Tailwind, ShadCN UI, and Velite, and deployed with Vercel. These frameworks allow the site to be statically rendered, leading to a site with a fast first contentful paint and less client-side rendering, improving the speed on the user's end.
Using MDX and Velite for articles creates a simple framework for writing articles, where markdown content that you may have used from note-taking apps like Obsidian or Notion can be quickly edited and published. By adding YAML frontmatter (less intimidating than it sounds) and renaming the file from .md
to .mdx
, you can drop the file into a folder within the project and push the changes to github, and voila, you have a published article. MDX also allows you to go much deeper with its ability to embed Javascript components within an article, like an embedded Youtube video or a demo of your project.
Editing the Forked Site
Once you have forked the github repository (tutorial here), open the project in your code editor. Get the project started by running npm i
to install the node packages for the site, and then npm run dev
to get the site running locally. Go to the address the site is hosted on so you can see the changes happening (this is likely localhost:3000).
We will first need to edit the file config/site.ts
to contain your information. Replace each of the fields with your own information, and add any links or text that will be repeated frequently in the site. Below is my site's config, with some different links and information from the original Jollyblog code.
export const siteConfig = {
name: "trow.dev",
url: "https://trow.dev",
description: "Data Analysis, Business Intelligence, Machine Learning",
author: "Trevor Rowland",
title: "Data Analyst",
links: {
email: "mailto:[email protected]",
github: "https://github.com/dBCooper2",
instagram: "https://instagram.com/trevor_r0", // Added from
linkedin: "https://www.linkedin.com/in/trevor-rowland711/",
},
};
export type SiteConfig = typeof siteConfig;
With these changes made, refresh the page on your site and you will see your information displayed. If this doesn't happen, you can stop (crtl+c
) the development server and restart it to make the changes appear.
Next, you can edit the about section to include your information. We can quickly change the headshot by replacing the image public/avatar.png
with your avatar image. Now navigate to app/about/page.tsx
and edit the file to include your info. Below is the content you need to edit.
export default async function AboutPage() {
return (
// Existing Code...
<div className="min-w-48 max-w-48 flex flex-col gap-2">
<Avatar className="h-48 w-48">
<AvatarImage src="/avatar.png" alt={siteConfig.author} />
<AvatarFallback>JC</AvatarFallback>
</Avatar>
<h2 className="text-2xl font-bold text-center break-words">
{siteConfig.author}
</h2>
<p className="text-muted-foreground text-center break-words">
Add your title here
</p>
</div>
<p className="text-muted-foreground text-lg py-4">
Add a Description Here
</p>
</div>
</div>
);
}
Now the last thing we need to change are the articles. The site should now have a homepage and about page with your information.
MDX Articles
Let's start with a basic article. To add an article to the site, we need to navigate to the content/blog/
folder and create a file, article-name.mdx
. Replace the title article-name
with what you want to appear in the URL, as this filename becomes the slug the site uses to navigate to your article.
Within this markdown file, add the following frontmatter to the top of your code. This is metadata the site uses to populate previews of each article as well as the headings on the article's page.
---
title: Add Your Title Here
description: Add a quick description here (This is optional!)
date: 2024-01-01 (Follow this format!)
tags: ["add", "topics", "that", "identify", "your", "article"]
published: true (This is true by default, and is also optional)
---
Once this is finished, add your writing to the document and check on the development server to make sure the article is rendering properly. If your article is purely text, this is a good enough stopping point. Your site is now set up, and you can skip to the extras and deployment section to get the site up and running.
Adding more to the MDX Articles
If you would like to add images, videos, and custom code to MDX, use the following examples to get this set up. For an image, you can embed it directly in the site with markdown with the following code (and you can add a caption as well).
Images in MDX
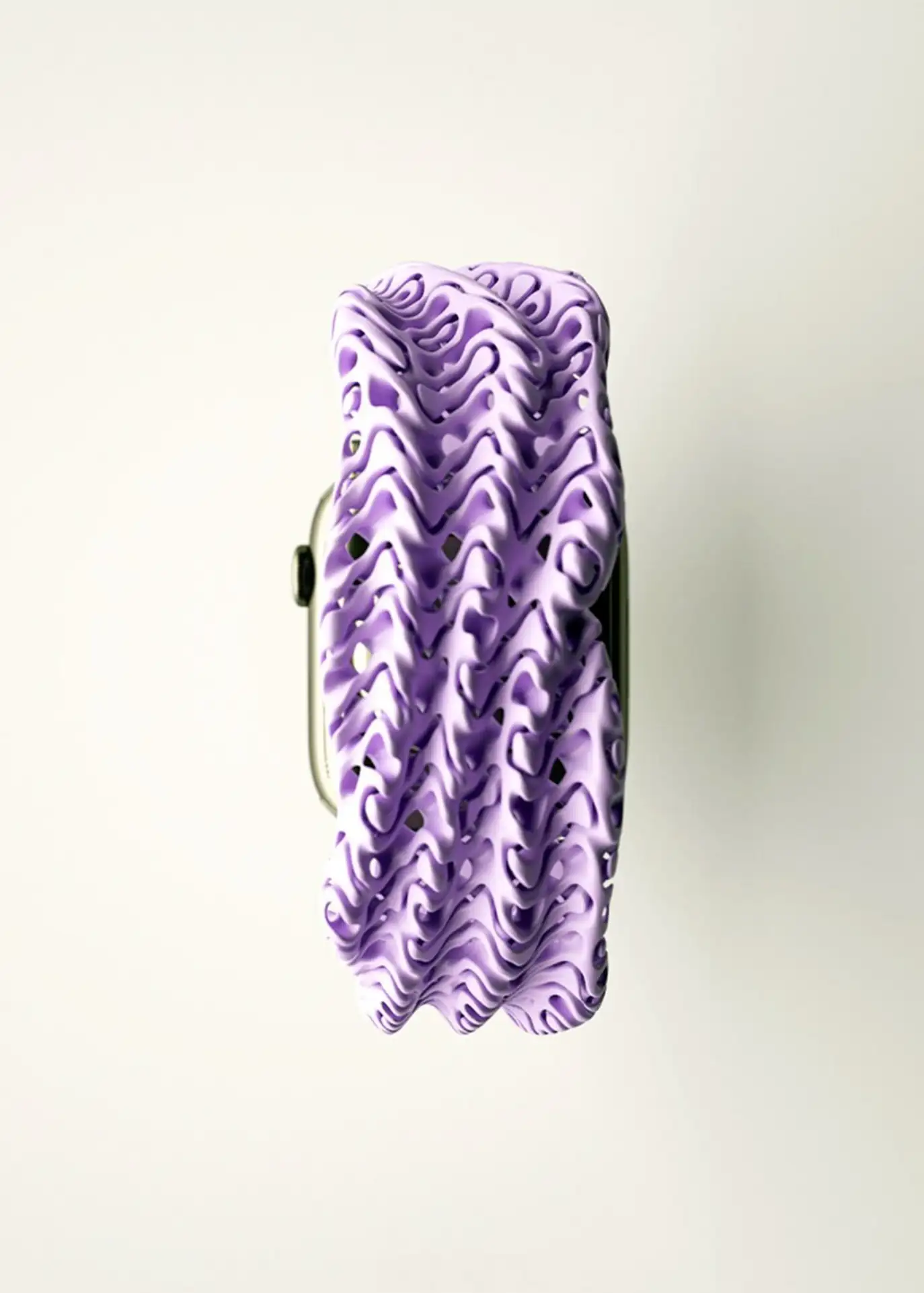
<Caption>[Unda Loop](<link-to-article>) by [Ross Lovegrove](<https://www.rosslovegrove.com/>)</Caption>
This searches for an image in the public/articles/2024-12-05/
directory. You can organize the folders in public/
however you want, this is just how I do it.
For custom code components like a video embed, you will need to add the code yourself. You can add the component to the components/
folder, and then add it to the components/mdx-components.tsx
file to make it available to articles. Add the component to the imports in this file, and then add the named component to the list of components in the file.
Custom Code Components in MDX
import Image from "next/image";
import Link from "next/link";
import * as runtime from "react/jsx-runtime";
import { cn } from "@/lib/utils";
import BoidSandbox from "./mdx/BoidSandbox"; // Here is the component I added
import BoidsVisualization from "./BoidsVisualization";
import { Card } from "./ui/card";
import { slug } from "github-slugger";
// Existing Code...
const components = {
Image,
Callout,
a: CustomLink,
BoidSandbox, //Here is the Component I added
BoidsVisualization,
Card,
Caption,
h1: (props: any) => <Heading level={1} {...props} />,
h2: (props: any) => <Heading level={2} {...props} />,
h3: (props: any) => <Heading level={3} {...props} />,
h4: (props: any) => <Heading level={4} {...props} />,
h5: (props: any) => <Heading level={5} {...props} />,
h6: (props: any) => <Heading level={6} {...props} />,
};
The sky is the limit with these custom code components. I want to shout out Josh W Comeau for his amazing use of this in his articles, check out his site to get a better idea of how powerful these components can be.
Extras
Now the site should be fully functional and displaying your articles. Let's quickly cover how to add different icons and favicons, change the site's theming, and changing the links across the site.
Color Changes
First, we will change the color theming of the website. I am not great at coming up with palettes yet, so to make this site's palette I pasted the globals.css
file into ChatGPT and asked it to change the colors to the themes I wanted. I then pasted the new globals.css
code into the file, and manually adjusted it until it looked how I wanted. The globals.css
file has a light and dark theme, so keep that in mind when you are picking the colors for your site.
Logos
If you navigate back to the homepage (localhost:3000
), you should see a 'dB' icon in the top left corner. This is an icon that I made into an SVG, and added to the project. To change this, take a PNG file you'd like to use as the logo for the site, and convert it into an SVG tag here. This SVG tag will be used in the file components/icons.tsx
. Simply replace the following section with your generated code (This can be done for any other logos you want to use as well).
export const Icons = {
logo: (props: IconProps) => (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="682.667"
height="682.667"
version="1"
viewBox="0 0 512 512"
fill="currentColor"
>
<path d="M173.6 143.2c-1.2 4.7-1.2 4.7 12.5 5 11.3.3 13.3.6 14.3 2 .8 1.4-1.3 11.3-9.8 45.5-13.2 53-12.5 50.3-13.6 50.3-.4 0-1.2-1.5-1.5-3.3-1.9-8.7-9.7-18.2-17.7-21.7-7.2-3.1-20.3-2.5-29.6 1.3-13.3 5.5-28.9 18.6-38.9 32.8-9.4 13.3-17.8 34.3-20.4 51.3-1.6 9.9-.6 27.5 2 35.7 8.1 25.7 32 36.6 55.1 25.3 5.9-2.9 9.6-5.8 17.3-13.5 6-6.1 9.7-9.2 9.7-8.1 0 .9-1.1 6-2.5 11.2s-2.5 9.8-2.5 10.2c0 .5 10.3.8 23 .8h22.9l.9-3.3.8-3.2-9.5-.5c-5.3-.3-10.2-.9-10.8-1.3-.7-.5-1.3-2.1-1.3-3.8 0-2.2 49.6-203.3 52.6-213.2.5-1.6-1.2-1.7-25.9-1.7h-26.5zm-15 87.4c9 5.1 14.2 19.1 13.1 34.8-.3 4.7-3 17.7-6.2 30.6-6.6 26.3-11.6 38.1-21.2 49.4-6.5 7.8-16.2 15-22.6 16.7-5.9 1.6-15.1.7-18.5-1.8-3.6-2.7-6.9-7.7-8.4-12.7-2.1-7.1-1.4-26.5 1.6-40.7 7.7-37.6 21.4-65.8 36.3-75 8.5-5.3 18.3-5.8 25.9-1.3M280.1 144.7c-.6 2.1-1.1 4.1-1.1 4.5 0 .5 4.7.8 10.4.8 12.8 0 16.3.7 18.2 3.5 1.3 2-.6 10.4-23.1 100.6-23.5 94-24.7 98.5-27.5 101.1-3 2.8-3.2 2.8-16.5 2.8h-13.4l-1 3.7c-.6 2.1-1.1 4.4-1.1 5 0 2 112.2 1.8 124.5-.2 37-6 64.1-28 72.6-59 5.8-21.3-2.4-41.1-21.2-51-3.8-2-9.7-4.2-13-4.9-3.3-.6-5.9-1.6-5.9-2.1.1-.6 2.9-1.8 6.2-2.7 14-3.9 28.6-12.8 38.9-23.5 11.4-11.9 17.1-24.7 17.2-38.8.1-11.6-2.7-18.1-11.2-26.5-7.6-7.6-15.9-12.1-27.5-15-7.5-1.9-11.2-2-66.2-2h-58.3zm118.7 8.8c19.8 8.9 23.5 31.5 9.7 60-8.1 16.9-20.3 26.8-36.2 29.4-4.2.7-16.3 1.1-31.4.9l-24.6-.3 10.7-43.2c5.9-23.7 11.4-44.3 12.4-45.7.9-1.4 2.9-2.8 4.4-3.2 1.5-.3 3.2-.8 3.7-1 .6-.2 10.9-.2 23-.1 21.7.3 22.1.4 28.3 3.2m-25.7 103.3c7.2 2.9 13.1 8 16.1 14 3.4 6.9 3.2 22.8-.6 36.5-6.3 23.4-16 36.9-32.2 45-9.8 4.9-15.3 5.7-40.1 5.7-25.2 0-26.7-.3-26.6-5.6.1-2.3 22.1-92.4 23.8-97.2.4-1.1 5.6-1.3 27.3-1 24.7.4 27.1.6 32.3 2.6"></path>
</svg>
),
Make sure you are only replacing the <svg>
tag and not the logo: (props: IconProps) => (
section. If the pasted svg is missing the {...props}
section, make sure you add that as well.
Favicon
The Favicon is an image that sits in the tab your website is in within the browser. You should see another "dB" logo in the tab your localhost:3000
instance is running in. To create a favicon, find an image you want to use and go to Favicon.io and follow the steps there. To add the code they want you to paste into the site's head, paste it into components/site-header.tsx
. Also replace all the icons and favicons in the app/
directory with the ones from Favicon.io.
More Social Media Links
To add something like an Instagram or BlueSky link that you defined in config/site.ts
, you will need to have the link in config/site.ts
, added an SVG logo to components/icons.tsx
, and then add the links to the site header, mobile nav and main nav. To do this, navigate to components/mobile-nav.tsx
. Here you should see how the other links are set up, and can follow their format to add your own links.
// Within the MobileNav() return statement...
<SheetContent side="right">
<MobileLink
onOpenChange={setOpen}
href="/"
className="flex items-center"
>
<Icons.logo className="mr-2 h-4 w-4" />
<span className="font-bold">{siteConfig.name}</span>
</MobileLink>
<div className="flex flex-col gap-3 mt-3">
<MobileLink onOpenChange={setOpen} href="/blog">
Blog
</MobileLink>
<MobileLink onOpenChange={setOpen} href="/about">
About
</MobileLink>
<Link target="_blank" rel="noreferrer" href={siteConfig.links.github}>
GitHub
</Link>
<Link
target="_blank"
rel="noreferrer"
href={siteConfig.links.twitter}
>
Twitter
</Link>
</div>
</SheetContent>
// Existing Code...
Now the extra features of the site are customized for your website. Check to make sure everything looks good in the development server before testing if the site is ready for deployment. Once this is done, stop the server and run the command npm run build
. This is just a check to make sure the site will properly deploy. Fix any errors that appear, then move on to deployment.
Deployment
My site is deployed using Vercel, and I have been happy with them so far. Their deployment service is incredibly simple. You connect a github repository, and Vercel will build and host the site.
Navigate to their website and create an account if you do not have one. All you'll need is a hobby account so don't pay for anything.
Next, you should find a page called 'Overview' for your account. Go to Add New…
and select Project. From here, sign into your github and give Vercel access to the website's repository. Then follow the steps to deploy the website, and you're all set!
Deployment: Domains
Vercel will give you a free domain to use, however I purchased my own, trow.dev through CloudFlare. You can also purchase a domain through vercel, but if you use cloudflare, follow this guide to get set up with it.
And now you should be done! Feel free to reach out with comments or criticism of the project, I want this to be a site that I can continually improve.